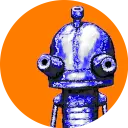
How to create QR Codes
Main tool to create qr codes is qrencode
# apt-get install qrencode
Relevant options are
- -o FILENAME, –output=FILENAME
- -s NUMBER, –size=NUMBER # specify the size of dot (pixel.
(default=3)
- -l {LMQH}, –level={LMQH} # specify error correction level from L
(lowest) to H (highest. (default=L)
- -t {PNG,PNG32,EPS,SVG,XPM,ANSI,ANSI256,ASCII,ASCIIi,UTF8,UTF8i,ANSIUTF8,ANSIUTF8i,ANSI256UTF8}. (default=PNG)
Create a QR Code for your wifi
The QR code standard that mobile devices understand comes in the following format:
WIFI:S:{SSID name of your network};T:{security type - WPA or WEP};P:{the network password};;
Special characters may have to be escaped using a “" character. So for example if your WiFi name was”MyWLAN” and your password was “publicsecretpassphrasemax63” using WPA encryption you would encode:
WIFI:S:MyWLAN;T:WPA;P:publicsecretpassphrasemax63;;
Create a QR Code for a camera device to import private SSH key
You generated pairs of SSH keys on your main computer and want to use SSH from your mobile device? Generate a QR Code and transfer the private key via camera!
cat id_key_ed25519| qrencode -l L -t UTF8
Here a little script to run each time you want to create a qr code for your wifi (sane), an URL (creepy) or a SSH key (?):
#!/bin/env bash
echo -e "Create a QR Code for
- an (U)RL,
- a (W)LAN connection
- a private (S)SH key file\n
For URLs and WLAN connections, find the .png in your home directory\n
The picture of the private key will NOT be stored\n"
function url {
echo "Enter URL"
read -p "> " URL
#regex='(^(ht|f)tp(s?)\:\/\/[0-9a-zA-Z]([-.\w]*[0-9a-zA-Z])*(:(0-9)*)*(\/?)([a-zA-Z0-9\-\.\?\,\'\/\\\+&%\$#_]*)?$")'
regex='(https?|ftp|file)://[-[:alnum:]\+&@#/%?=~_|!:,.;]+'
if [[ $URL =~ $regex ]]
then
domain=$(echo $URL | tr -c [:alnum:] '.' | tr -s '.')
echo $domain
qrencode -s 5 -l H -o $HOME/$domain.png $URL
else
echo "$URL is not a valid URL"
fi
}
function wifi {
echo "Enter SSID"
read -p "> " SSID
echo "Enter passphrase"
read -p "> " PASSPHRASE
qrencode -s 5 -l H -o $HOME/$SSID.png "WIFI:S:${SSID};T:WPA;P:${PASSPHRASE};;"
}
function ssh {
echo "Select a private key file"
n=0
for i in $(ls $HOME/.ssh/id_* |grep -v .pub$)
do
n=$((n+1))
printf "$n - ${i##*/}\n"
eval "i${n}=\$i"
done
if [ "$n" -eq 0 ]
then
echo >&2 No private key found.
exit
fi
printf 'Enter ID (1 to %s): ' "$n"
read -p "> " num
num=$(printf '%s\n' "$num" | tr -dc '[:digit:]')
if [ "$num" -le 0 ] || [ "$num" -gt "$n" ]
then
echo >&2 Wrong selection.
exit 1
else
eval "i=\$i${num}"
cat "$i"| qrencode -l L -t UTF8
fi
}
read -p "> " UWS
case $UWS in
u|U|url|URL)
url
;;
w|W|WIFI|wifi)
wifi
;;
s|S|ssh|SSH)
ssh
;;
esac
exit