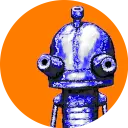
BASH
Press space to continue
read -n1 -r -p "Press any key to continue..." key
The -n1 specifies that it only waits for a single
character.
The -r puts it into raw mode, which is necessary because
otherwise, if you press something like backslash, it doesn’t register
until you hit the next key.
The -p specifies the prompt, which must be quoted if it
contains spaces.
The key argument is only necessary if you want to know which
key they pressed, in which case you can access it through
$key.
If you are using Bash, you can also specify a timeout with -t, which causes read to return a failure when a key isn’t pressed. So for example:
read -t5 -n1 -r -p 'Press any key in the next five seconds...' key
if [ "$?" -eq "0" ]; then
echo 'A key was pressed.'
else
echo 'No key was pressed.'
fi
read without any parameters will only continue if you press enter. Use read –n1 if you want to continue if any key is pressed.
If you just need to pause a loop or script, and you’re happy to press enter instead of any key, then read on its own will do the job.
do_stuff
read
do_more_stuff
How can I use parameter expansion? How can I get substrings?
Parameter Expansion substitutes a variable or special parameter for its value. It is the primary way of dereferencing (referring to) variables in Bourne-like shells such as Bash. Parameter expansion can also perform various operations on the value at the same time for convenience. Remember to quote your expansions.
The first set of capabilities involves removing a substring, from either the beginning or the end of a parameter. Here’s an example using parameter expansion with something akin to a hostname (dot-separated components):
parameter | result |
---|---|
$name | polish.ostrich.racing.champion |
${name#*.} | ostrich.racing.champion |
${name##*.} | champion |
${name%%.*} | polish |
${name%.*} | polish.ostrich.racing |
And here’s an example of the parameter expansions for a typical filename:
parameter | result |
---|---|
$file | /usr/share/java-1.4.2-sun/demo/applets/Clock/Clock.class |
${file#*/} | usr/share/java-1.4.2-sun/demo/applets/Clock/Clock.class |
${file##*/} | Clock.class |
${file%%/*} | |
${file%/*} | /usr/share/java-1.4.2-sun/demo/applets/Clock |
US keyboard users may find it helpful to observe that, on the
keyboard, the “#” is to the left of the “%” symbol.
Mnemonically, “#” operates on the left side of a parameter, and “%”
operates on the right.
The glob after the “%” or “%%” or “#” or “##” specifies what pattern to
remove from the parameter expansion.
Another mnemonic is that in an English sentence “#” usually comes
before a number (e.g., “The #1 Bash reference site”),
while “%” usually comes after a number (e.g., “Now 5% discounted”), so
they operate on those sides.
You cannot nest parameter expansions. If you need to perform two expansions steps, use a variable to hold the result of the first expansion:
# foo holds: key="some value"
bar=${foo#*=\"} bar=${bar%\"*}
# now bar holds: some value
Redirection: In the shell, what does ” 2>&1 ” mean?
- File descriptor 0 is the standard input (stin).
- File descriptor 1 is the standard output (stdout).
- File descriptor 2 is the standard error (stderr).
Here is one way to remember this construct (although it is not entirely accurate): at first, 2>1 may look like a good way to redirect stderr to stdout. However, it will actually be interpreted as “redirect stderr to a file named 1”. & indicates that what follows and precedes is a file descriptor and not a filename. So the construct becomes: 2>&1. Consider >& as redirect merger operator.
Syntax command |& … could be used as an alias for command 2>&1 | …. Same rules about command line order applies.
For redirecting both output from a given command, we see that a right syntax could be:
$ command >/dev/null 2>&1
for this special case, there is a shortcut syntax: &> … or >&
$ command &>/dev/null
$ command >&/dev/null